Adding Custom Options to React Native Developer Menu
TLDR: You can customize the Developer Menu in React Native with your custom actions. If you're already familiar with the Developer Menu, click here to jump to section about customizing the menu
What is The Developer Menu
When running a development version of a React Native app, you can access a special Developer Menu by either shaking your device (if you're running the app on the device instead of simular) or by using the following keyboard shortcuts:
- iOS Cmd ⌘ + D
- Android Cmd ⌘ + M
Doing either of these commands or shaking the device (by device I mean your phone, don't shake your laptop) should reveal menu that looks like this:
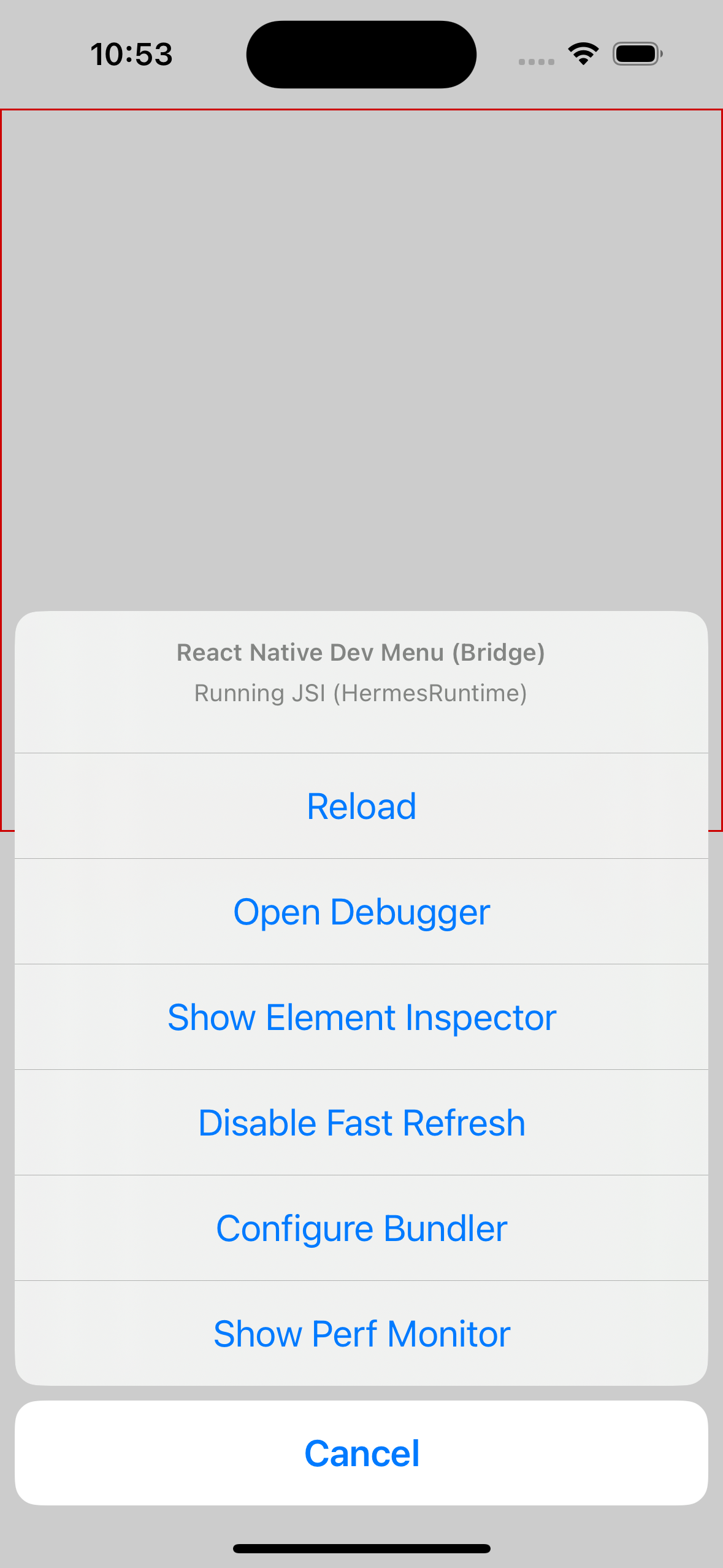
Here's a what all of these items in the list do
-
Reload
Refreshes the application by loading the latest JavaScript code bundled by the Metro Bundler. -
Open Debugger
Opens a new browser tab for debugging JavaScript code using Chrome's developer tools. -
Open React DevTools
Opens React DevTools for debugging the app's React component hierarchy. -
Show Inspector
Opens an inspection tool for examining and modifying the layout and style of React Native components. -
Show Inspector
Allows inspection of UI elements by tapping on them to see detailed information. -
Disable Fast Refresh
Disables the Fast Refresh feature, which automatically reloads the app upon saving code changes. -
Configure Bundler
Provides options to configure the Metro Bundler used in the React Native app. -
Show Perf Monitor
Displays a performance monitor with real-time stats like FPS and CPU usage.
Customizing The Developer Menu
You can also add your own items to the Developer menu. React Native comes bundled with The DevSettings module which exposes methods for customizing the options in the menu.
Import DevSettings
from react-native
:
import { DevSettings } from 'react-native'
Call the static addMenuItem()
in the part of the code you want. It takes two required parameters, title
string and handler
function. The title is used also as a key, so it needs to be unique for every item you add:
DevSettings.addMenuItem('My custom action 1', () => {
console.log('Custom action pressed')
})
DevSettings.addMenuItem('My custom action 2', () => {
console.log('Custom action pressed')
})
You can add as many items as you want into the Developer Menu as the list is scrollable. I personally like to wrap the addMenuItem()
calls inside __DEV__
check so that they only work in dev mode (the developer menu is not included in production builds in any case so this is in a way just for readability, say for example if a person not familiar with React Native is wondering where this code goes.):
if (__DEV__) {
DevSettings.addMenuItem('My custom action 1', () => {
console.log('Custom action pressed')
})
}